If the anonymous class instance doesn’t call any method, nor read or write any property of the container class, then it wouldn’t retain it.
By my understanding, all inner classes hold implicit reference to its container class object, so how to understand the above statements (if the anonymous class doesn’t access to container object’s method or property, then the inner class doesn’t retain the container class’s reference)
Hi @rainrain ! Thanks for your question.
An anonymous class won’t hold a reference to the container class if you don’t call any method nor access any property of the container class.
Have a look at the following example:
interface MyInterface {
fun callMe()
}
class MyClass {
private val myProperty = "This is a test"
fun method() {
val myInterface = object : MyInterface {
override fun callMe() {
println(myProperty)
}
}
myInterface.callMe()
}
}
fun main() {
val myClass = MyClass()
myClass.method()
}
If you put a breakpoint where you execute myInterface.callMe()
you’ll see the following:
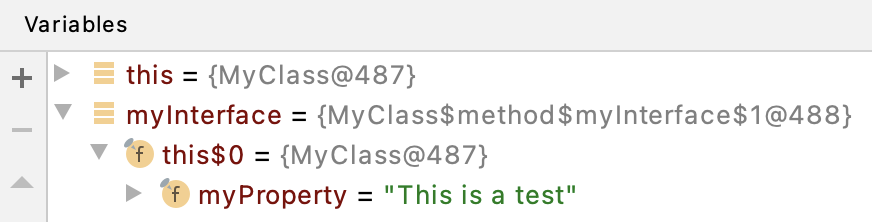
As you can see, you’re retaining the container class because you’re accessing myProperty
.
Now, if you change the code to print a text (instead of the property):
...
override fun callMe() {
println("This is a test")
}
...
And debug again, you’ll see the following:
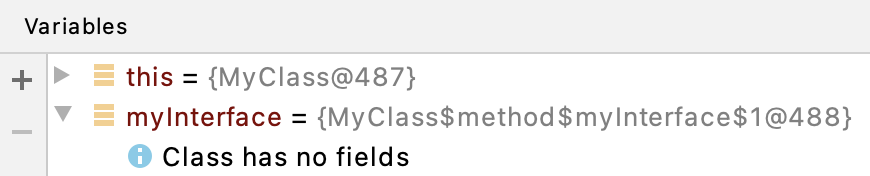
As you can see it’s not retaining the container class anymore.
1 Like